How To Program Hunter Node
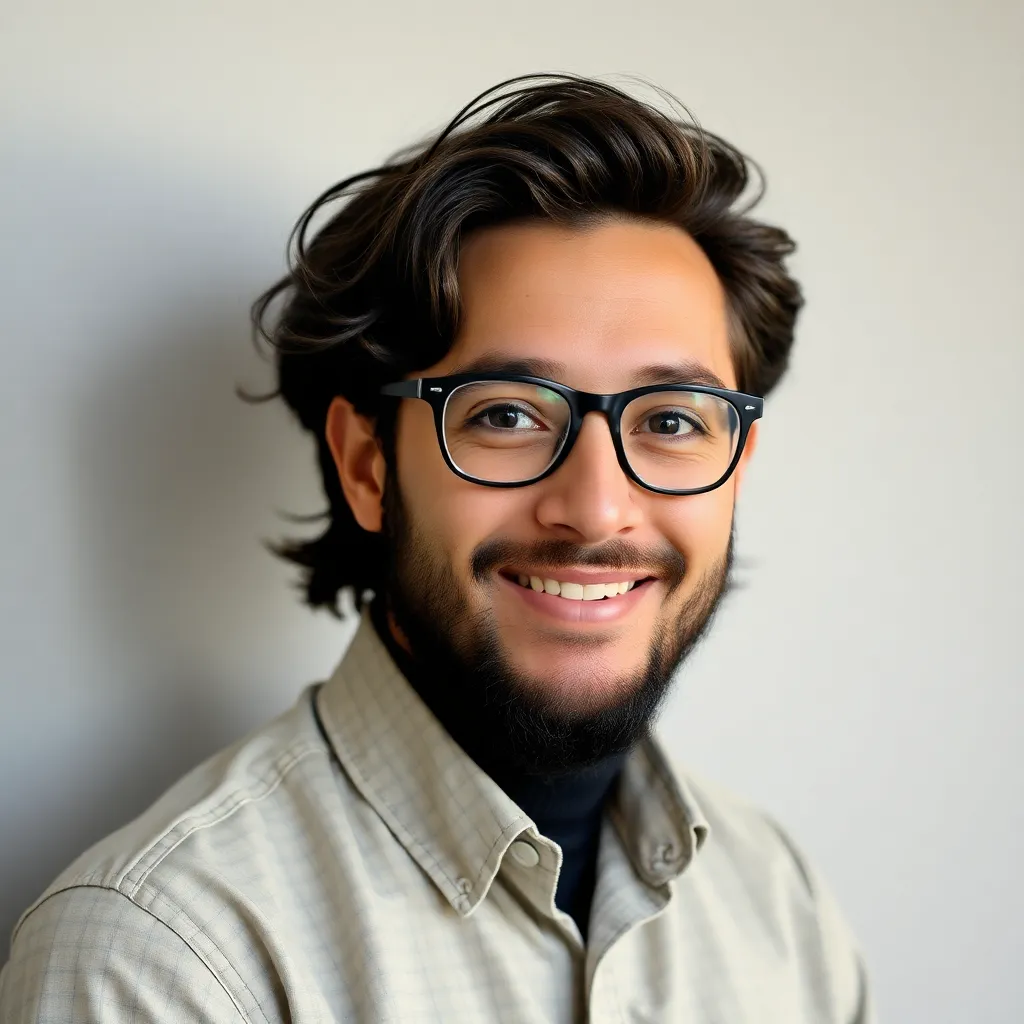
Ronan Farrow
Apr 15, 2025 · 3 min read

Table of Contents
How to Program a Hunter Node
Hunter nodes, while not a standard term in mainstream programming, likely refer to a custom-built node within a larger system designed for specific tasks like data acquisition or network reconnaissance. This guide will help you understand the fundamental concepts involved in building such a node, assuming a general familiarity with programming and networking.
Understanding the Hunter Node Concept
Before diving into the code, let's clarify the purpose of a "hunter node." It acts as a decentralized, independent unit within a network, actively seeking and collecting information. Think of it as a robotic scout, autonomously exploring and reporting its findings back to a central system. Its programming focuses on three key aspects:
- Target Identification: Defining what the hunter node should search for. This could involve specific IP addresses, file types, network signatures, or even patterns in data streams.
- Data Acquisition: The methods used to collect the target information. This might include network scanning, data scraping, file downloads, or sensor readings.
- Data Transmission: The mechanism for securely relaying the gathered information back to a central server or database. This often involves encryption and robust error handling.
Essential Programming Elements
The specific programming language used will depend on the project's needs and the developer's preferences. Python, with its extensive libraries for networking and data manipulation, is a popular choice. Here's a breakdown of the crucial elements:
1. Network Programming
- Sockets: Fundamental for establishing connections and communication with other network devices. You'll use libraries like
socket
in Python to create and manage sockets. - Protocols: Understanding relevant protocols (TCP/IP, UDP, HTTP) is essential for interacting with different network services and devices.
- Scanning Techniques: If your hunter node involves network scanning, you'll need to implement techniques like ping sweeps, port scans, or vulnerability scans using libraries like
scapy
ornmap
. Important Note: Always obtain explicit permission before scanning networks that you do not own. Unauthorized scanning is illegal and unethical.
2. Data Acquisition & Processing
- Data Parsing: Once the hunter node collects data, it often needs to be processed and structured. This might involve parsing XML, JSON, or other data formats. Libraries like
xml.etree.ElementTree
orjson
in Python are helpful here. - Data Cleaning: Handling inconsistencies or errors in the data is critical to ensure accuracy. This step involves techniques like data validation, filtering, and transformation.
- Regular Expressions: Regular expressions are powerful tools for pattern matching and extracting specific information from text-based data. Python's
re
module provides robust regex functionality.
3. Secure Data Transmission
- Encryption: Encrypting the gathered data is crucial to protect its confidentiality during transmission. Libraries like
cryptography
in Python provide tools for encryption and decryption. - Authentication: Verifying the identity of the hunter node and the central server is vital to prevent unauthorized access. Methods like SSL/TLS certificates are commonly used.
- Error Handling: Implementing robust error handling is essential to prevent data loss and ensure reliable communication. This involves managing exceptions, retries, and logging errors for debugging.
Example Code Snippet (Conceptual)
This is a simplified Python example demonstrating the core concept of connecting to a server and transmitting data:
import socket
# ... (Network configuration and encryption setup) ...
try:
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
sock.connect(('server_ip', server_port))
sock.sendall(encrypted_data) # Send the acquired data
response = sock.recv(1024) # Receive a response from the server
print(f"Server Response: {response.decode()}")
except Exception as e:
print(f"Error: {e}")
finally:
sock.close()
Security Considerations
Building a secure hunter node is paramount. Always adhere to ethical and legal guidelines. Consider these points:
- Minimize attack surface: Use only necessary libraries and services.
- Regular updates: Keep your software and libraries up-to-date to patch vulnerabilities.
- Strong authentication: Implement robust authentication and authorization mechanisms.
- Data sanitization: Carefully sanitize and validate all incoming data to prevent injection attacks.
This guide provides a foundational understanding. Remember to thoroughly research and understand the specifics of your target environment and legal implications before developing and deploying your hunter node. Always prioritize security and ethical considerations.
Featured Posts
Also read the following articles
Article Title | Date |
---|---|
How To Start A School Bus Company In Nj | Apr 15, 2025 |
How To Repair Cracked John Deere Hood | Apr 15, 2025 |
How To Stack Hay On A Wagon | Apr 15, 2025 |
How To Remove Rust Stains From A Pool | Apr 15, 2025 |
How To Pray For Someone Who Is Depressed | Apr 15, 2025 |
Latest Posts
Thank you for visiting our website which covers about How To Program Hunter Node . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.